Validation Rules
Validation rules can be used to ensure that the values that end users have entered into a field are valid and should let the user continue with their submission.
When a validation rule fails, feedback will be provided to the user that there is an issue with the fields value.
Validation rules have the following common settings:
Setting Name | Description |
---|---|
Rule Name | Name for the validation rule that is used in the admin panel and status windows. |
Override Validation Message | Validation rules have rule specific validation messages when they fail. If you wish to override these default messages that are returned to the user then use this setting. |
Required
The rule will fail if no value has been provided.
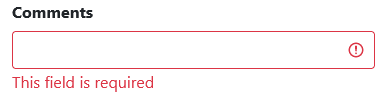
Regex
The rule will fail if the regex doesn’t match.
If there is no value then the regex rule will pass. If you want to force at least some input then combine this with a required rule.
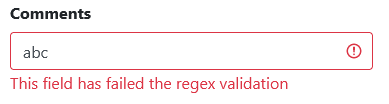
Sample Regex’s:
Description | Regex | Suggested Override Message |
---|---|---|
4 Numbers |
| Must contain exactly 4 numbers |
Email Address |
| Must enter a valid email address |
Australian Post Code |
| Must be a valid Australian post code |
Custom
The custom validation rule allows you to write JavaScript that returns true or false.
The rule will fail if the JavaScript returns false, null or fails to execute.
There are three variables that can be accessed:
Variable | Description |
---|---|
| Current value of the field being validated. |
| Field Id of the field being validated. |
| Container that contains the field being validated. |
Example JavaScript to check value in current field equals another field with id “test”:
var targetFieldId = "test"
var targetField = window.store.getters["form/getters/field/find"](targetFieldId);
if (targetField) {
if (targetField.value[0] == fieldValue[0]){
return true;
}
} else {
console.log("Couldn't locate target field")
}
return false;
Query Validator
The query validator can be used to execute a query against one of the supported providers.
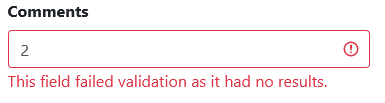
The validating fields current value can be accessed by the following placeholder:
Placeholder | Description |
---|---|
| Returns the current value of the field being validated. |
Other field values are able to be referenced via the standard fields placeholder.
When using the following providers please note the following:
ODBC/SQL/Authority Server
The query returning no rows will make the validation rule fail.
The query returning one row with multiple columns will make the validation rule pass.
The query returning one row with a single column will evaluate the following:
A value of empty string or null will make the validation rule fail.
A value of “false” will make the validation rule fail.
A value of “true” will make the validation rule pass.
Any other value will make the validation rule pass.
Sample Queries:
Description | Query |
---|---|
Check if a work order number has already been processed. This query returns ‘false’ if 1 or more matching rows are found and ‘true’ if there are none. |
CODE
|
Check if a work order number exists. This query will return the whole row if a match is found. |
CODE
|
EzeScan WebApps Placeholders
The placeholder returning the string “false” will make the validation rule fail.
The placeholder returning the string “true” will make the validation rule pass.
The placeholder returning an empty value will make the validation rule fail.
The placeholder returning a value that is not “false” will make the validation rule pass.
The placeholder returning a false boolean will make the validation fail.
Sample Placeholders:
Description | Placeholder |
---|---|
Field value equals “123” |
|
Field value starts with “INV” |
|
Field value greater than “5” |
|
Field value equals the value of a field with id “other_field” |
|
Connector Validator
The connector validator can be used to execute a query against one of our supported integrations via our connectors.
The validating fields current value can be accessed by the following placeholder:
Placeholder | Description |
---|---|
| Returns the current value of the field being validated. |
Other field values are able to be referenced via the standard fields placeholder.